#!/usr/bin/env python
# coding: utf-8
#
#
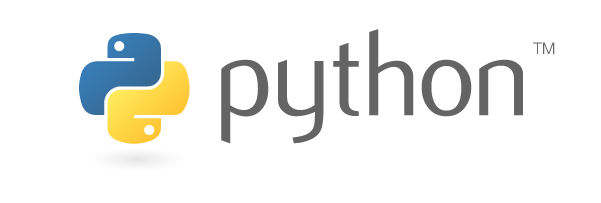
#
#
# PYTHON
# ==
#
# Python is an interpreted programming language oriented to easy-readable coding, unlike compiled languages like C/C++ and Fortran, where the syntax usually does not favor the readability. This feature makes Python very interesting when we want to focus on something different than the program structure itself, e.g. on Computational Methods, thereby allowing to optimize our time, to debug syntax errors easily, etc.
#
#
# [Official page](https://www.python.org/)
#
# [Wikipedia](http://en.wikipedia.org/wiki/Python)
#
# Python Philosophy
# --
# 1. Beautiful is better than ugly.
# 2. Explicit is better than implicit.
# 3. Simple is better than complex.
# 4. Complex is better than complicated.
# 5. Flat is better than nested.
# 6. Sparse is better than dense.
# 7. Readability counts.
# 8. Special cases aren't special enough to break the rules. (Although practicality beats purity)
# 9. Errors should never pass silently. (Unless explicitly silenced)
# 10. In the face of ambiguity, refuse the temptation to guess.
# 11. There should be one-- and preferably only one --obvious way to do it. (Although that way may not be obvious at first unless you're Dutch)
# 12. Now is better than never. (Although never is often better than right now)
# 13. If the implementation is hard to explain, it's a bad idea.
# 14. If the implementation is easy to explain, it may be a good idea.
# 15. NameSpaces are one honking great idea -- let's do more of those!
# - - -
# - [Hello World!](#Hello-World!)
# - [Arithmetics](#Arithmetics)
# - [Lists, Tuples and Dictionaries](#Lists,-Tuples-and-Dictionaries)
# - [Bucles and Conditionals](#Bucles-and-Conditionals)
# - [Functions](#Functions)
# - - -
# # Hello World!
# In[1]:
print('Hello World!')
# And also allows scripting: *(This code should be copied on a file 'hello.py')*
# In[2]:
#! /usr/bin/python
#This is a comment
print('Hello World!')
# # Arithmetics
# ## Sum
# In[3]:
5.89+4.89
# ## Multiplication
# In[4]:
120*4.5
# ## **Division**
# In[5]:
#Unless you use decimals, python does not support complete division (only integer division)
#This only applies for Python 2.X
100/3
# In[6]:
100/3.
# ## **Power**
# In[7]:
2**6
# ## **Module**
# In[8]:
10%2
# In[9]:
20%3
# ## **Scientific notation**
# In[10]:
(1.0e24/3. + 2.9e23)/1e-2
# # Lists, Tuples and Dictionaries
# ## Lists
# Lists are useful when you want to store and manipulate a set of elements (even of different types).
# In[31]:
#A list is declared using [] and may content different type of objects
lista = ["abc", 42, 3.1415]
print lista
# In[32]:
#First element of the list
lista[0]
# In[33]:
#Last element of the list
lista[-1]
# In[34]:
#Adding a new element (boolean element)
lista.append(True)
print lista
# In[35]:
#Inserting a new second element
lista.insert(1, "I am second :)")
print lista
# In[36]:
#Deleting the third element of the list
del lista[3]
print lista
# In[37]:
#Reassign the first element of the list
lista[0] = "xyz"
print lista
# In[40]:
#Showing the elements from 0 to 2
lista[0:3]
# In[41]:
#Showing elements two by two
lista[::2]
# In[25]:
#It is possible to embed a list
embedded_list = [lista, [True, 42L]]
print embedded_list
# In[26]:
#Second element of the first list
embedded_list[0][1]
# In[44]:
#A matrix as a list of embedded lists
A = [ [1,2], [3,4] ]
print A
# In[46]:
#When two list are added, the result is a new concatenated list
[1,2,"ab",True,[1,2]] + [3.1415,"Pi","circle"]
# ## Tuples
# A tuple is almost equal to a list, except that once declared its elements, it is not possible to modify them. Therefore, tuples are useful when you only want to store some elements but not modify them.
# In[ ]:
#A tuple is declared using ()
tupla = ("abc", 42, 3.1415)
print tupla
# In[65]:
#It is not possible to add more elements
tupla.append("xy")
# In[66]:
#It is not possible to delete an element
del tupla[0]
# In[67]:
#It is not possible to modify an existing element
tupla[0] = "xy"
# ## Dictionaries
# Dictionaries are extremely useful when manipulating complex data.
# In[69]:
#A dictionary is declared using {}, and specifying the name of the component, then the character : followed by the element to store.
dictionary = { "Colombia":"Bogota", "Argentina":"Buenos Aires", "Bolivia":"La Paz", "Francia":"Paris", "Alemania":"Berlin" }
print dictionary
#Note the order in a dictionary does not matter as one identifies a single element through a string, not a number
# In[71]:
#Instead of a number, an element of a dictionary is accessed with the name of the component
print dictionary["Colombia"]
print dictionary["Alemania"]
# In[2]:
#The elements of the dictionary may be of any type
dictionary2 = { "Enteros":[1,2,3,4,5], "Ciudad":"Medellin", "Cedula":1128400433, "Colores":["Amarillo", "Azul", "Rojo"] }
print dictionary2["Colores"][1]
# In[3]:
#The elements of the dictionary can be modified only by changing directly such an element
dictionary2["Ciudad"] = "Bogota"
print dictionary2
# In[4]:
#Adding a new element is possible by only defining the new component
dictionary2["Pais"] = "Colombia"
print dictionary2
# In[5]:
#The command del can be also used for deleting an element, as a list
del dictionary2["Colores"]
print dictionary2
# # Bucles and Conditionals
# ## if
# Conditionals are useful when we want to check some condition.
# The statements `elif` and `else` can be used when more than one condition is possible or when there is something to do when condition is not fulfilled.
# In[16]:
x = 10
y = 2
if x > 5 and y==2:
print "True"
# In[29]:
x = 4
y = 3
if x>5 or y<2:
print "True 1"
elif x==4:
print "True 2"
else:
print "False"
# ## for
# `For` cycles are specially useful when we want to sweep a set of elements with a known size.
# In[22]:
for i in xrange(0,5,1):
print i, i**2
# In[24]:
suma = 0
for i in xrange(0,10,1):
suma += i**2
print "The result is %d"%(suma)
# In[25]:
for language in ['Python', 'C', 'C++', 'Ruby', 'Java']:
print language
# In[51]:
serie = [ i**2 for i in xrange(1,10) ]
print( serie )
# ## while
# `While` cycles are specially useful when we want to sweep a set of elements with an unknown size.
# In[33]:
#! /usr/bin/python
number = int(input("Write a positive number: "))
while numero < 0:
print("You wrote a negative number. Do it again")
numero = int(input("Write a positive number: "))
print("Thank you!")
# In[40]:
from numpy.random import random
x = 0
while x<0.9:
x = random()
print x
print "The selected number was", x
# # Functions
# ## **Explicit functions**
# In[74]:
def f(x,y):
return x*y
f(3,2)
# In[75]:
#It is possible to assign default arguments
def f(x,y=2):
return x*y
#When evaluating, we can omit the default argument
f(3)
# In[80]:
#It is possible to specify explicitly the order of the arguments
def f(x,y):
return x**y
print 'f(1,2)=',f(1,2)
print 'f(2,1)=',f(y=1,x=2)
# ## Implicit functions
# Implicit functions are usdeful when we want to use a function once.
# In[82]:
f = lambda x,y: x**y
f(3,2)
# In[85]:
#It is possible to pass functions as arguments of other function
def f2( f, x ):
return f(x)**2
#We can define a new function explicitly
def f(x):
return x+2
print "Explicit: f(2)^2 =", f2(f,2)
#Or define the function implicitly
print "Implicit: f(2)^2 =", f2(lambda x:x+2,2)